Here is another version of the script that correctly produces the colored .obj file.
const jscad = require('@jscad/modeling')
const { polygon } = jscad.primitives
const { union } = jscad.booleans
const { extrudeLinear } = jscad.extrusions
const { colorNameToRgb } = jscad.colors
function getParameterDefinitions() {
return [
{ name: 'larg', type: 'int', initial: 100, caption: 'largeur ?' },
{ name: 'haut', type: 'int', initial: 100, caption: 'Hauteur?' },
{ name: 'prof', type: 'int', initial: 10, caption: 'Profondeur ?' },
{ name: 'ep', type: 'int', initial: 10, caption: 'Epaisseur ?' }
]
}
const main = (params) => {
let larg = params.larg
let haut = params.haut
let prof = params.prof
let ep = params.ep
let pExt = []
let x = larg/2
let y = haut/2
pExt.push ([x, y])
pExt.push ([x, -y])
pExt.push ([-x, -y])
pExt.push ([-x, y])
let pInt = []
x = larg/2 - ep
y = haut/2 - ep
pInt.push ([x, y])
pInt.push ([x, -y])
pInt.push ([-x, -y])
pInt.push ([-x, y])
let R = []
for (let i = 0; i < 4; i++) {
let i2 = i < 3 ? i+1 : 0
R.push(polygon({points: [pExt[i], pInt[i], pInt[i2], pExt[i2]]}));
}
let C2d = union(R)
let C3d = extrudeLinear({height: prof}, C2d)
console.log(C3d)
const cols = [ "blue", "yellow", "red", "green" ]
let pc = new Array(32)
pc.fill(0,0,16)
pc.fill(1,0,2)
pc.fill(1,4,6)
pc.fill(1,8,10)
pc.fill(1,12,14)
pc.fill(2,16,24)
pc.fill(3,24,32)
C3d.polygons.forEach((P, index) => P.color = colorNameToRgb(cols[pc[index]]))
return C3d
}
module.exports = { getParameterDefinitions, main }
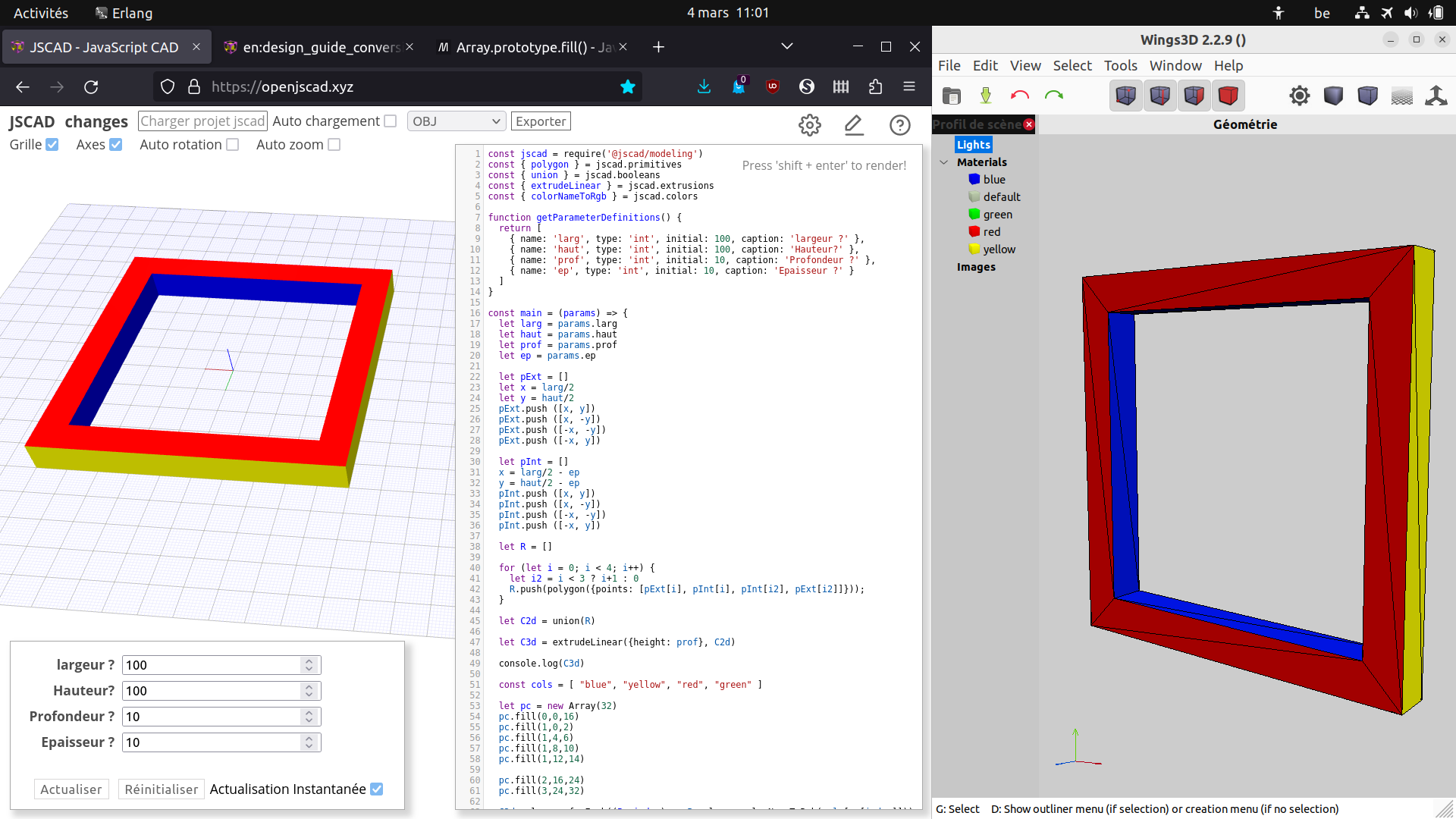
The coloring code could be improved, instead of manual inputs I could test polygons values.